mirror of
https://github.com/letic/terraform-provider-google.git
synced 2024-09-07 09:36:44 +00:00
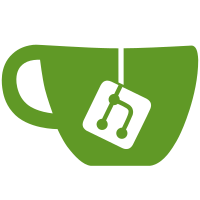
Switch to using Go modules. This migrates our vendor.json to use Go 1.11's modules system, and replaces the vendor folder with the output of go mod vendor. The vendored code should remain basically the same; I believe some tree shaking of packages and support scripts/licenses/READMEs/etc. happened. This also fixes Travis and our Makefile to no longer use govendor.
37 lines
1.1 KiB
Go
37 lines
1.1 KiB
Go
package hcldec
|
|
|
|
import (
|
|
"github.com/hashicorp/hcl2/hcl"
|
|
)
|
|
|
|
// Variables processes the given body with the given spec and returns a
|
|
// list of the variable traversals that would be required to decode
|
|
// the same pairing of body and spec.
|
|
//
|
|
// This can be used to conditionally populate the variables in the EvalContext
|
|
// passed to Decode, for applications where a static scope is insufficient.
|
|
//
|
|
// If the given body is not compliant with the given schema, the result may
|
|
// be incomplete, but that's assumed to be okay because the eventual call
|
|
// to Decode will produce error diagnostics anyway.
|
|
func Variables(body hcl.Body, spec Spec) []hcl.Traversal {
|
|
var vars []hcl.Traversal
|
|
schema := ImpliedSchema(spec)
|
|
content, _, _ := body.PartialContent(schema)
|
|
|
|
if vs, ok := spec.(specNeedingVariables); ok {
|
|
vars = append(vars, vs.variablesNeeded(content)...)
|
|
}
|
|
|
|
var visitFn visitFunc
|
|
visitFn = func(s Spec) {
|
|
if vs, ok := s.(specNeedingVariables); ok {
|
|
vars = append(vars, vs.variablesNeeded(content)...)
|
|
}
|
|
s.visitSameBodyChildren(visitFn)
|
|
}
|
|
spec.visitSameBodyChildren(visitFn)
|
|
|
|
return vars
|
|
}
|