mirror of
https://github.com/letic/terraform-provider-google.git
synced 2024-07-09 11:38:29 +00:00
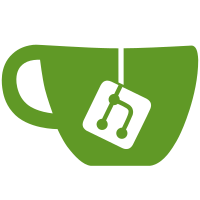
Switch to using Go modules. This migrates our vendor.json to use Go 1.11's modules system, and replaces the vendor folder with the output of go mod vendor. The vendored code should remain basically the same; I believe some tree shaking of packages and support scripts/licenses/READMEs/etc. happened. This also fixes Travis and our Makefile to no longer use govendor.
58 lines
1.8 KiB
Go
58 lines
1.8 KiB
Go
package cleanhttp
|
|
|
|
import (
|
|
"net"
|
|
"net/http"
|
|
"runtime"
|
|
"time"
|
|
)
|
|
|
|
// DefaultTransport returns a new http.Transport with similar default values to
|
|
// http.DefaultTransport, but with idle connections and keepalives disabled.
|
|
func DefaultTransport() *http.Transport {
|
|
transport := DefaultPooledTransport()
|
|
transport.DisableKeepAlives = true
|
|
transport.MaxIdleConnsPerHost = -1
|
|
return transport
|
|
}
|
|
|
|
// DefaultPooledTransport returns a new http.Transport with similar default
|
|
// values to http.DefaultTransport. Do not use this for transient transports as
|
|
// it can leak file descriptors over time. Only use this for transports that
|
|
// will be re-used for the same host(s).
|
|
func DefaultPooledTransport() *http.Transport {
|
|
transport := &http.Transport{
|
|
Proxy: http.ProxyFromEnvironment,
|
|
DialContext: (&net.Dialer{
|
|
Timeout: 30 * time.Second,
|
|
KeepAlive: 30 * time.Second,
|
|
DualStack: true,
|
|
}).DialContext,
|
|
MaxIdleConns: 100,
|
|
IdleConnTimeout: 90 * time.Second,
|
|
TLSHandshakeTimeout: 10 * time.Second,
|
|
ExpectContinueTimeout: 1 * time.Second,
|
|
MaxIdleConnsPerHost: runtime.GOMAXPROCS(0) + 1,
|
|
}
|
|
return transport
|
|
}
|
|
|
|
// DefaultClient returns a new http.Client with similar default values to
|
|
// http.Client, but with a non-shared Transport, idle connections disabled, and
|
|
// keepalives disabled.
|
|
func DefaultClient() *http.Client {
|
|
return &http.Client{
|
|
Transport: DefaultTransport(),
|
|
}
|
|
}
|
|
|
|
// DefaultPooledClient returns a new http.Client with similar default values to
|
|
// http.Client, but with a shared Transport. Do not use this function for
|
|
// transient clients as it can leak file descriptors over time. Only use this
|
|
// for clients that will be re-used for the same host(s).
|
|
func DefaultPooledClient() *http.Client {
|
|
return &http.Client{
|
|
Transport: DefaultPooledTransport(),
|
|
}
|
|
}
|