mirror of
https://github.com/letic/terraform-provider-google.git
synced 2024-09-07 17:46:43 +00:00
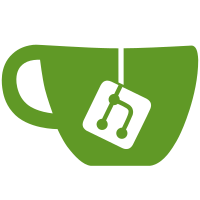
Switch to using Go modules. This migrates our vendor.json to use Go 1.11's modules system, and replaces the vendor folder with the output of go mod vendor. The vendored code should remain basically the same; I believe some tree shaking of packages and support scripts/licenses/READMEs/etc. happened. This also fixes Travis and our Makefile to no longer use govendor.
100 lines
3.1 KiB
Go
100 lines
3.1 KiB
Go
// Code generated by private/model/cli/gen-api/main.go. DO NOT EDIT.
|
|
|
|
package s3
|
|
|
|
import (
|
|
"github.com/aws/aws-sdk-go/aws"
|
|
"github.com/aws/aws-sdk-go/aws/client"
|
|
"github.com/aws/aws-sdk-go/aws/client/metadata"
|
|
"github.com/aws/aws-sdk-go/aws/request"
|
|
"github.com/aws/aws-sdk-go/aws/signer/v4"
|
|
"github.com/aws/aws-sdk-go/private/protocol/restxml"
|
|
)
|
|
|
|
// S3 provides the API operation methods for making requests to
|
|
// Amazon Simple Storage Service. See this package's package overview docs
|
|
// for details on the service.
|
|
//
|
|
// S3 methods are safe to use concurrently. It is not safe to
|
|
// modify mutate any of the struct's properties though.
|
|
type S3 struct {
|
|
*client.Client
|
|
}
|
|
|
|
// Used for custom client initialization logic
|
|
var initClient func(*client.Client)
|
|
|
|
// Used for custom request initialization logic
|
|
var initRequest func(*request.Request)
|
|
|
|
// Service information constants
|
|
const (
|
|
ServiceName = "s3" // Name of service.
|
|
EndpointsID = ServiceName // ID to lookup a service endpoint with.
|
|
ServiceID = "S3" // ServiceID is a unique identifer of a specific service.
|
|
)
|
|
|
|
// New creates a new instance of the S3 client with a session.
|
|
// If additional configuration is needed for the client instance use the optional
|
|
// aws.Config parameter to add your extra config.
|
|
//
|
|
// Example:
|
|
// // Create a S3 client from just a session.
|
|
// svc := s3.New(mySession)
|
|
//
|
|
// // Create a S3 client with additional configuration
|
|
// svc := s3.New(mySession, aws.NewConfig().WithRegion("us-west-2"))
|
|
func New(p client.ConfigProvider, cfgs ...*aws.Config) *S3 {
|
|
c := p.ClientConfig(EndpointsID, cfgs...)
|
|
return newClient(*c.Config, c.Handlers, c.Endpoint, c.SigningRegion, c.SigningName)
|
|
}
|
|
|
|
// newClient creates, initializes and returns a new service client instance.
|
|
func newClient(cfg aws.Config, handlers request.Handlers, endpoint, signingRegion, signingName string) *S3 {
|
|
svc := &S3{
|
|
Client: client.New(
|
|
cfg,
|
|
metadata.ClientInfo{
|
|
ServiceName: ServiceName,
|
|
ServiceID: ServiceID,
|
|
SigningName: signingName,
|
|
SigningRegion: signingRegion,
|
|
Endpoint: endpoint,
|
|
APIVersion: "2006-03-01",
|
|
},
|
|
handlers,
|
|
),
|
|
}
|
|
|
|
// Handlers
|
|
svc.Handlers.Sign.PushBackNamed(v4.BuildNamedHandler(v4.SignRequestHandler.Name, func(s *v4.Signer) {
|
|
s.DisableURIPathEscaping = true
|
|
}))
|
|
svc.Handlers.Build.PushBackNamed(restxml.BuildHandler)
|
|
svc.Handlers.Unmarshal.PushBackNamed(restxml.UnmarshalHandler)
|
|
svc.Handlers.UnmarshalMeta.PushBackNamed(restxml.UnmarshalMetaHandler)
|
|
svc.Handlers.UnmarshalError.PushBackNamed(restxml.UnmarshalErrorHandler)
|
|
|
|
svc.Handlers.UnmarshalStream.PushBackNamed(restxml.UnmarshalHandler)
|
|
|
|
// Run custom client initialization if present
|
|
if initClient != nil {
|
|
initClient(svc.Client)
|
|
}
|
|
|
|
return svc
|
|
}
|
|
|
|
// newRequest creates a new request for a S3 operation and runs any
|
|
// custom request initialization.
|
|
func (c *S3) newRequest(op *request.Operation, params, data interface{}) *request.Request {
|
|
req := c.NewRequest(op, params, data)
|
|
|
|
// Run custom request initialization if present
|
|
if initRequest != nil {
|
|
initRequest(req)
|
|
}
|
|
|
|
return req
|
|
}
|