mirror of
https://github.com/letic/terraform-provider-google.git
synced 2024-07-03 08:42:39 +00:00
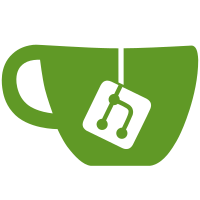
Previously, provider credentials were _supposed_ to be able to be specified as the file contents or the path to the file. We even had a test for the code for this! Then we updated the validation for the provider, and forgot to validate filepaths as ok. So provider validation failed. And because our test only tested the config validation, and not the provider validation, our tests thought this was just fine still. This fixes that oversight, accepting filepaths as valid. It also adds tests to ensure that provider validation allows both file paths and contents.
170 lines
4.2 KiB
Go
170 lines
4.2 KiB
Go
package google
|
|
|
|
import (
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
"strings"
|
|
"testing"
|
|
|
|
"github.com/hashicorp/terraform/helper/schema"
|
|
"github.com/hashicorp/terraform/terraform"
|
|
)
|
|
|
|
var testAccProviders map[string]terraform.ResourceProvider
|
|
var testAccProvider *schema.Provider
|
|
|
|
var credsEnvVars = []string{
|
|
"GOOGLE_CREDENTIALS",
|
|
"GOOGLE_CLOUD_KEYFILE_JSON",
|
|
"GCLOUD_KEYFILE_JSON",
|
|
"GOOGLE_USE_DEFAULT_CREDENTIALS",
|
|
}
|
|
|
|
var projectEnvVars = []string{
|
|
"GOOGLE_PROJECT",
|
|
"GCLOUD_PROJECT",
|
|
"CLOUDSDK_CORE_PROJECT",
|
|
}
|
|
|
|
var regionEnvVars = []string{
|
|
"GOOGLE_REGION",
|
|
"GCLOUD_REGION",
|
|
"CLOUDSDK_COMPUTE_REGION",
|
|
}
|
|
|
|
var orgEnvVars = []string{
|
|
"GOOGLE_ORG",
|
|
}
|
|
|
|
var billingAccountEnvVars = []string{
|
|
"GOOGLE_BILLING_ACCOUNT",
|
|
}
|
|
|
|
func init() {
|
|
testAccProvider = Provider().(*schema.Provider)
|
|
testAccProviders = map[string]terraform.ResourceProvider{
|
|
"google": testAccProvider,
|
|
}
|
|
}
|
|
|
|
func TestProvider(t *testing.T) {
|
|
if err := Provider().(*schema.Provider).InternalValidate(); err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
}
|
|
|
|
func TestProvider_impl(t *testing.T) {
|
|
var _ terraform.ResourceProvider = Provider()
|
|
}
|
|
|
|
func testAccPreCheck(t *testing.T) {
|
|
if v := os.Getenv("GOOGLE_CREDENTIALS_FILE"); v != "" {
|
|
creds, err := ioutil.ReadFile(v)
|
|
if err != nil {
|
|
t.Fatalf("Error reading GOOGLE_CREDENTIALS_FILE path: %s", err)
|
|
}
|
|
os.Setenv("GOOGLE_CREDENTIALS", string(creds))
|
|
}
|
|
|
|
if v := multiEnvSearch(credsEnvVars); v == "" {
|
|
t.Fatalf("One of %s must be set for acceptance tests", strings.Join(credsEnvVars, ", "))
|
|
}
|
|
|
|
if v := multiEnvSearch(projectEnvVars); v == "" {
|
|
t.Fatalf("One of %s must be set for acceptance tests", strings.Join(projectEnvVars, ", "))
|
|
}
|
|
|
|
if v := multiEnvSearch(regionEnvVars); v != "us-central1" {
|
|
t.Fatalf("One of %s must be set to us-central1 for acceptance tests", strings.Join(regionEnvVars, ", "))
|
|
}
|
|
}
|
|
|
|
func TestProvider_getRegionFromZone(t *testing.T) {
|
|
expected := "us-central1"
|
|
actual := getRegionFromZone("us-central1-f")
|
|
if expected != actual {
|
|
t.Fatalf("Region (%s) did not match expected value: %s", actual, expected)
|
|
}
|
|
}
|
|
|
|
func TestProvider_loadCredentialsFromFile(t *testing.T) {
|
|
ws, es := validateCredentials(testFakeCredentialsPath, "")
|
|
if len(ws) != 0 {
|
|
t.Errorf("Expected %d warnings, got %v", len(ws), ws)
|
|
}
|
|
if len(es) != 0 {
|
|
t.Errorf("Expected %d errors, got %v", len(es), es)
|
|
}
|
|
}
|
|
|
|
func TestProvider_loadCredentialsFromJSON(t *testing.T) {
|
|
contents, err := ioutil.ReadFile(testFakeCredentialsPath)
|
|
if err != nil {
|
|
t.Fatalf("Unexpected error: %s", err)
|
|
}
|
|
ws, es := validateCredentials(string(contents), "")
|
|
if len(ws) != 0 {
|
|
t.Errorf("Expected %d warnings, got %v", len(ws), ws)
|
|
}
|
|
if len(es) != 0 {
|
|
t.Errorf("Expected %d errors, got %v", len(es), es)
|
|
}
|
|
}
|
|
|
|
// getTestRegion has the same logic as the provider's getRegion, to be used in tests.
|
|
func getTestRegion(is *terraform.InstanceState, config *Config) (string, error) {
|
|
if res, ok := is.Attributes["region"]; ok {
|
|
return res, nil
|
|
}
|
|
if config.Region != "" {
|
|
return config.Region, nil
|
|
}
|
|
return "", fmt.Errorf("%q: required field is not set", "region")
|
|
}
|
|
|
|
// getTestProject has the same logic as the provider's getProject, to be used in tests.
|
|
func getTestProject(is *terraform.InstanceState, config *Config) (string, error) {
|
|
if res, ok := is.Attributes["project"]; ok {
|
|
return res, nil
|
|
}
|
|
if config.Project != "" {
|
|
return config.Project, nil
|
|
}
|
|
return "", fmt.Errorf("%q: required field is not set", "project")
|
|
}
|
|
|
|
// testAccPreCheck ensures at least one of the project env variables is set.
|
|
func getTestProjectFromEnv() string {
|
|
return multiEnvSearch(projectEnvVars)
|
|
}
|
|
|
|
// testAccPreCheck ensures at least one of the credentials env variables is set.
|
|
func getTestCredsFromEnv() string {
|
|
return multiEnvSearch(credsEnvVars)
|
|
}
|
|
|
|
// testAccPreCheck ensures at least one of the region env variables is set.
|
|
func getTestRegionFromEnv() string {
|
|
return multiEnvSearch(regionEnvVars)
|
|
}
|
|
|
|
func getTestOrgFromEnv(t *testing.T) string {
|
|
skipIfEnvNotSet(t, orgEnvVars...)
|
|
return multiEnvSearch(orgEnvVars)
|
|
}
|
|
|
|
func getTestBillingAccountFromEnv(t *testing.T) string {
|
|
skipIfEnvNotSet(t, billingAccountEnvVars...)
|
|
return multiEnvSearch(billingAccountEnvVars)
|
|
}
|
|
|
|
func multiEnvSearch(ks []string) string {
|
|
for _, k := range ks {
|
|
if v := os.Getenv(k); v != "" {
|
|
return v
|
|
}
|
|
}
|
|
return ""
|
|
}
|