mirror of
https://github.com/letic/terraform-provider-google.git
synced 2024-09-07 01:26:43 +00:00
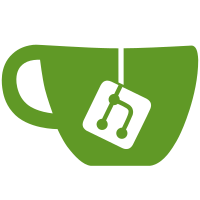
Switch to using Go modules. This migrates our vendor.json to use Go 1.11's modules system, and replaces the vendor folder with the output of go mod vendor. The vendored code should remain basically the same; I believe some tree shaking of packages and support scripts/licenses/READMEs/etc. happened. This also fixes Travis and our Makefile to no longer use govendor.
75 lines
1.7 KiB
Go
75 lines
1.7 KiB
Go
package hclwrite
|
|
|
|
import (
|
|
"github.com/hashicorp/hcl2/hcl/hclsyntax"
|
|
"github.com/zclconf/go-cty/cty"
|
|
)
|
|
|
|
type Block struct {
|
|
inTree
|
|
|
|
leadComments *node
|
|
typeName *node
|
|
labels nodeSet
|
|
open *node
|
|
body *node
|
|
close *node
|
|
}
|
|
|
|
func newBlock() *Block {
|
|
return &Block{
|
|
inTree: newInTree(),
|
|
labels: newNodeSet(),
|
|
}
|
|
}
|
|
|
|
// NewBlock constructs a new, empty block with the given type name and labels.
|
|
func NewBlock(typeName string, labels []string) *Block {
|
|
block := newBlock()
|
|
block.init(typeName, labels)
|
|
return block
|
|
}
|
|
|
|
func (b *Block) init(typeName string, labels []string) {
|
|
nameTok := newIdentToken(typeName)
|
|
nameObj := newIdentifier(nameTok)
|
|
b.leadComments = b.children.Append(newComments(nil))
|
|
b.typeName = b.children.Append(nameObj)
|
|
for _, label := range labels {
|
|
labelToks := TokensForValue(cty.StringVal(label))
|
|
labelObj := newQuoted(labelToks)
|
|
labelNode := b.children.Append(labelObj)
|
|
b.labels.Add(labelNode)
|
|
}
|
|
b.open = b.children.AppendUnstructuredTokens(Tokens{
|
|
{
|
|
Type: hclsyntax.TokenOBrace,
|
|
Bytes: []byte{'{'},
|
|
},
|
|
{
|
|
Type: hclsyntax.TokenNewline,
|
|
Bytes: []byte{'\n'},
|
|
},
|
|
})
|
|
body := newBody() // initially totally empty; caller can append to it subsequently
|
|
b.body = b.children.Append(body)
|
|
b.close = b.children.AppendUnstructuredTokens(Tokens{
|
|
{
|
|
Type: hclsyntax.TokenCBrace,
|
|
Bytes: []byte{'}'},
|
|
},
|
|
{
|
|
Type: hclsyntax.TokenNewline,
|
|
Bytes: []byte{'\n'},
|
|
},
|
|
})
|
|
}
|
|
|
|
// Body returns the body that represents the content of the receiving block.
|
|
//
|
|
// Appending to or otherwise modifying this body will make changes to the
|
|
// tokens that are generated between the blocks open and close braces.
|
|
func (b *Block) Body() *Body {
|
|
return b.body.content.(*Body)
|
|
}
|