mirror of
https://github.com/letic/terraform-provider-google.git
synced 2024-07-23 18:16:01 +00:00
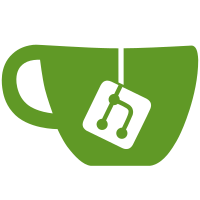
Switch to using Go modules. This migrates our vendor.json to use Go 1.11's modules system, and replaces the vendor folder with the output of go mod vendor. The vendored code should remain basically the same; I believe some tree shaking of packages and support scripts/licenses/READMEs/etc. happened. This also fixes Travis and our Makefile to no longer use govendor.
52 lines
1.2 KiB
Go
52 lines
1.2 KiB
Go
package multierror
|
|
|
|
import (
|
|
"fmt"
|
|
)
|
|
|
|
// Error is an error type to track multiple errors. This is used to
|
|
// accumulate errors in cases and return them as a single "error".
|
|
type Error struct {
|
|
Errors []error
|
|
ErrorFormat ErrorFormatFunc
|
|
}
|
|
|
|
func (e *Error) Error() string {
|
|
fn := e.ErrorFormat
|
|
if fn == nil {
|
|
fn = ListFormatFunc
|
|
}
|
|
|
|
return fn(e.Errors)
|
|
}
|
|
|
|
// ErrorOrNil returns an error interface if this Error represents
|
|
// a list of errors, or returns nil if the list of errors is empty. This
|
|
// function is useful at the end of accumulation to make sure that the value
|
|
// returned represents the existence of errors.
|
|
func (e *Error) ErrorOrNil() error {
|
|
if e == nil {
|
|
return nil
|
|
}
|
|
if len(e.Errors) == 0 {
|
|
return nil
|
|
}
|
|
|
|
return e
|
|
}
|
|
|
|
func (e *Error) GoString() string {
|
|
return fmt.Sprintf("*%#v", *e)
|
|
}
|
|
|
|
// WrappedErrors returns the list of errors that this Error is wrapping.
|
|
// It is an implementation of the errwrap.Wrapper interface so that
|
|
// multierror.Error can be used with that library.
|
|
//
|
|
// This method is not safe to be called concurrently and is no different
|
|
// than accessing the Errors field directly. It is implemented only to
|
|
// satisfy the errwrap.Wrapper interface.
|
|
func (e *Error) WrappedErrors() []error {
|
|
return e.Errors
|
|
}
|