mirror of
https://github.com/letic/terraform-provider-google.git
synced 2024-07-03 08:42:39 +00:00
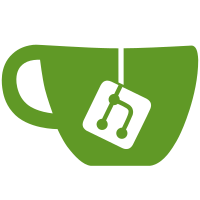
Add consistency for for IAM imports. - Adds imports for projects, folders, crypto keys, organizations, and key rings. - Anything else with IAM can implement a simple method and begin working immediately. - Add tests for all the IAM imports. - Import documentation for IAM resources.
58 lines
1.5 KiB
Go
58 lines
1.5 KiB
Go
package google
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
|
|
"github.com/hashicorp/terraform/helper/acctest"
|
|
"github.com/hashicorp/terraform/helper/resource"
|
|
)
|
|
|
|
func TestAccGoogleOrganizationIamMember_importBasic(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
orgId := getTestOrgFromEnv(t)
|
|
account := acctest.RandomWithPrefix("tf-test")
|
|
projectId := getTestProjectFromEnv()
|
|
|
|
resource.Test(t, resource.TestCase{
|
|
PreCheck: func() { testAccPreCheck(t) },
|
|
Providers: testAccProviders,
|
|
Steps: []resource.TestStep{
|
|
resource.TestStep{
|
|
Config: testAccGoogleOrganizationIamMember_basic(account, orgId),
|
|
},
|
|
|
|
resource.TestStep{
|
|
ResourceName: "google_organization_iam_member.foo",
|
|
ImportStateId: fmt.Sprintf("%s roles/browser serviceAccount:%s@%s.iam.gserviceaccount.com", orgId, account, projectId),
|
|
ImportState: true,
|
|
},
|
|
},
|
|
})
|
|
}
|
|
|
|
func TestAccGoogleOrganizationIamBinding_importBasic(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
orgId := getTestOrgFromEnv(t)
|
|
account := acctest.RandomWithPrefix("tf-test")
|
|
roleId := "tfIamTest" + acctest.RandString(10)
|
|
|
|
resource.Test(t, resource.TestCase{
|
|
PreCheck: func() { testAccPreCheck(t) },
|
|
Providers: testAccProviders,
|
|
Steps: []resource.TestStep{
|
|
resource.TestStep{
|
|
Config: testAccGoogleOrganizationIamBinding_basic(account, roleId, orgId),
|
|
},
|
|
|
|
resource.TestStep{
|
|
ResourceName: "google_organization_iam_binding.foo",
|
|
ImportStateId: fmt.Sprintf("%s organizations/%s/roles/%s", orgId, orgId, roleId),
|
|
ImportState: true,
|
|
},
|
|
},
|
|
})
|
|
}
|